Couchbase Golang "failed to get query provider: not connected to cluster | {"statement":"*"}"
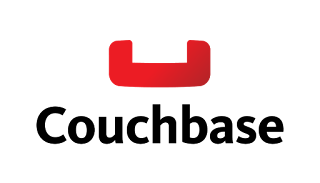
Go (also known as Golang) is an open source programming language developed by Google Couchbase is a NoSQL database built for business-critical applications. While using Golang sdk 2.0 for Couchbase, I encountered an error "failed to get query provider: not connected to cluster | {"statement":"*"}" But this error had a strange behaviour, whenever I run any insert or update operation via Sdk function prior running Cluster. Query (query, &gocb.QueryOptions{NamedParameters: params}) this error didn't occurred. Running Just Cluster. Query (query, &gocb.QueryOptions{NamedParameters: params}) after connection directly would get "failed to get query provider: not connected to cluster | {"statement":"*"}" What I did to tackle this is, prior to the Query function or immediately after running the connect function I ran a atomic function collection. Binary (). Increment ( "serverUp"